Introduction
Web developers often need to analyze how websites load to optimize performance and enhance user experience. Chrome DevTools provides powerful features to examine this process in detail.
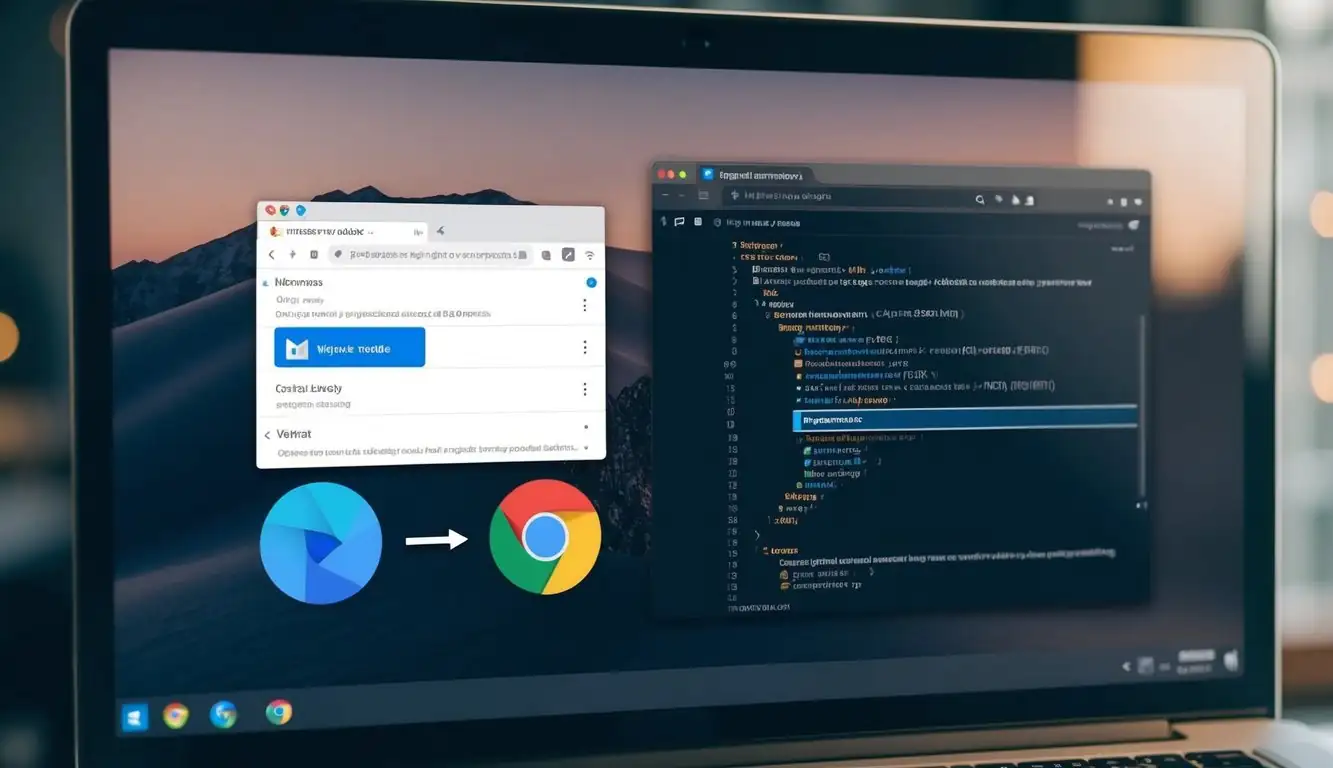
We can use Chrome's developer tools to watch a website load step by step and pause loading at specific points. This allows us to inspect the page state, examine network requests, and debug JavaScript execution at any moment during the loading process.
By leveraging Chrome DevTools' Sources panel, we can pause script execution and analyze the code line by line. This granular control enables us to identify performance bottlenecks, troubleshoot rendering issues, and gain valuable insights into how our websites behave during loading.
Understanding Chrome DevTools
Chrome DevTools is a powerful set of web development tools built directly into the Google Chrome browser. It offers a wide array of features for debugging, optimizing, and analyzing websites.
Discovering the Features of Chrome DevTools
Chrome DevTools provides an extensive toolkit for web developers. We can use it to inspect and modify HTML, CSS, and JavaScript in real-time. The Console panel allows us to run JavaScript code and view logged messages.
The Network panel helps us analyze network requests and responses, enabling performance optimization. For debugging, we have access to breakpoints, watch expressions, and call stack information. The Application panel lets us inspect storage, service workers, and manifest files.
Chrome DevTools also includes advanced features like the Performance panel for profiling page load and runtime performance. The Security panel helps identify potential security issues on websites.
Navigating the DevTools Interface
To access Chrome DevTools, we can right-click anywhere on a webpage and select "Inspect" or use the keyboard shortcut Ctrl+Shift+I (Windows/Linux) or Cmd+Option+I (Mac).
The DevTools interface is divided into panels, each focusing on different aspects of web development. The Elements panel is where we can inspect and modify the DOM and CSS. The Sources panel is useful for debugging JavaScript.
We can customize the DevTools layout by dragging panels to rearrange them. The settings menu (gear icon) allows us to adjust preferences and enable experimental features. The Command Menu (Ctrl+Shift+P or Cmd+Shift+P) provides quick access to various DevTools functions.
Setting Up Your Environment
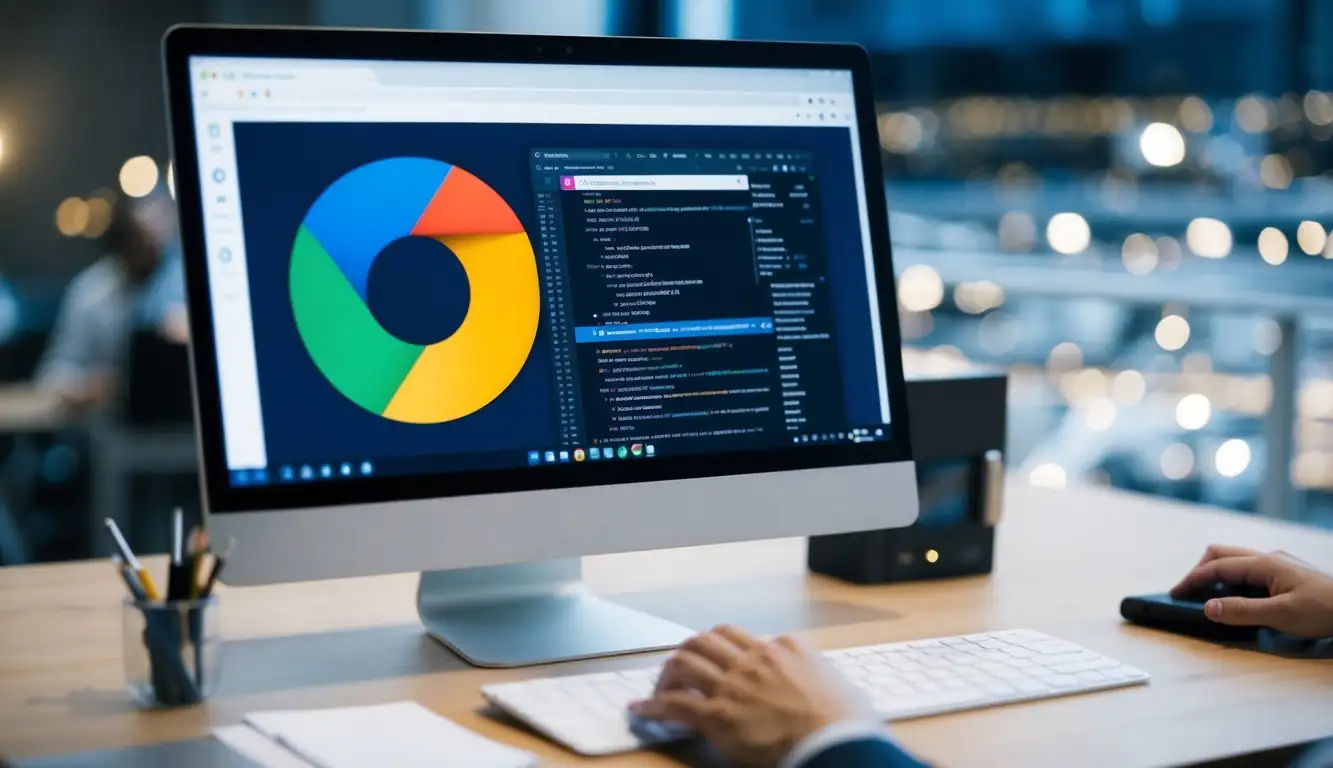
Proper configuration of Chrome DevTools is essential for effective website analysis. We'll explore how to enable and customize DevTools to optimize your workflow.
Enabling DevTools in Chrome
To access Chrome DevTools, we press F12 or Ctrl+Shift+I (Cmd+Option+I on Mac) while in the Chrome browser. Alternatively, we right-click anywhere on a webpage and select "Inspect" from the context menu. DevTools opens as a panel within the browser window.
We can also open DevTools from the Chrome menu . Click the three-dot menu in the top right corner, go to "More tools," and select "Developer tools."
DevTools offers various panels, including Elements, Console, Sources, and Network. Each panel provides specific tools for inspecting and debugging web pages.
Customizing the DevTools Panels
Chrome DevTools allows extensive customization to suit our preferences. We access the settings by clicking the gear icon in the top right corner of the DevTools window.
In the settings, we can:
- Choose a light or dark theme
- Dock DevTools to different positions (right, bottom, or separate window)
- Enable experimental features
We customize panel layouts by dragging and dropping tabs. To focus on specific tasks, we hide unused panels by right-clicking on the tab bar and deselecting them.
Chrome's DevTools offers extensions for additional functionality. We install these from the Chrome Web Store to enhance our debugging capabilities.
Inspecting the Document Object Model (DOM)
The DOM represents the structure of a web page. We can use Chrome DevTools to explore and manipulate DOM elements in real-time, providing valuable insights for debugging and development.
Exploring the Elements Panel
The Elements panel in Chrome DevTools is our gateway to DOM inspection. We can view and change the DOM easily here. To access it, we right-click on any element and select "Inspect" or press Ctrl+Shift+I (Windows) or Cmd+Option+I (Mac).
In the Elements panel, we see a tree-like structure of DOM elements. We can expand and collapse nodes to navigate through the page structure. Hovering over elements highlights them on the page.
To edit an element, we double-click on it. We can modify text content, attributes, and even add or remove elements. These changes are reflected instantly on the page.
Monitoring DOM Changes
Chrome DevTools allows us to track DOM modifications in real-time. This feature is invaluable for debugging dynamic content and JavaScript interactions.
To break on DOM changes, we right-click an element in the Elements panel and select "Break on" followed by the type of change we want to monitor. Options include subtree modifications, attribute modifications, and node removal.
When a watched DOM change occurs, the debugger pauses execution. We can then step through the code to understand what's causing the modification. This helps us pinpoint exactly where and why DOM changes are happening.
Analyzing CSS and Layout Shifts
Chrome DevTools provides powerful features for examining CSS styles and identifying layout shifts. These tools help developers optimize website performance and user experience.
Investigating CSS Styles
In Chrome DevTools, we can inspect and modify CSS styles in real-time. Open the Elements tab and select an element to view its applied styles. The Styles pane shows all CSS rules affecting the element, including inherited styles and user agent stylesheets.
Use the filter box to search for specific properties. We can toggle individual properties on and off to see their impact. To edit a value, double-click it and enter a new one. Chrome DevTools also allows us to add new CSS rules directly in the browser.
The Computed tab displays the final computed styles for an element after all CSS rules have been applied. This view is particularly useful for understanding inheritance and specificity.
Identifying and Fixing Layout Shifts
Layout shifts can significantly impact user experience. The Performance tab in Chrome DevTools helps us identify and analyze these shifts. Record a page load and look for red blocks in the Experience section, which indicate layout shifts.
Cumulative Layout Shift (CLS) measures the total amount of unexpected layout shifts. To reduce CLS, we can:
- Set explicit dimensions for images and embedded elements
- Reserve space for dynamic content
- Use CSS transform for animations instead of properties that trigger layout changes
The Sources tab allows us to pause script execution at specific points, helping isolate the cause of layout shifts. By combining these tools, we can effectively diagnose and fix layout issues, improving overall website stability.
Debugging JavaScript
Debugging JavaScript is crucial for identifying and fixing issues in web applications. We'll explore how to use Chrome DevTools to debug JavaScript effectively, focusing on the Console Panel and managing breakpoints.
Utilizing the Console Panel
The Console Panel is a powerful tool for debugging JavaScript. We can use console.log() to output variable values and debug information. This method helps us track the flow of our code and identify potential issues.
To access the Console, we open Chrome DevTools and select the Console tab. Here, we can enter JavaScript commands directly or view logged messages from our code.
A console.log() is versatile. We can log simple strings, variables, or even complex objects:
console.log("Hello, debugging world!");
console.log(myVariable);
console.log({name: "John", age: 30});
The Console also displays errors and warnings, making it easier to spot issues in our code.
Managing JavaScript Breakpoints
Breakpoints allow us to pause code execution at specific points, examining the state of our application. Chrome DevTools offers several types of breakpoints.
To set a line-of-code breakpoint, we open the Sources panel, locate our JavaScript file, and click on the line number where we want to pause execution. When the code reaches this point, it will stop, allowing us to inspect variables and step through the code.
We can also set conditional breakpoints that only trigger when a specific condition is met. This is useful for debugging issues that occur under certain circumstances.
Event listener breakpoints are another powerful tool. They pause execution when specific events, like clicks or key presses, occur. This helps us debug event-driven code more effectively.
Leveraging Breakpoints for Debugging
Breakpoints are powerful tools for pausing code execution and inspecting the state of your web application. We'll explore different types of breakpoints in Chrome DevTools and how to use them effectively for debugging.
Setting Up Line-of-Code Breakpoints
Line-of-code breakpoints allow us to pause execution at specific points in our JavaScript code. To set one, we open the Sources panel in Chrome DevTools and click the line number where we want to pause.
When the breakpoint is hit, we can examine variables, step through code, and analyze the call stack. This is particularly useful for debugging complex logic or unexpected behavior.
We can also use conditional breakpoints by right-clicking the line number and entering a condition. The code will only pause if the condition is true, helping us target specific scenarios.
Handling XHR and Fetch Breakpoints
XHR and Fetch breakpoints are invaluable for debugging network requests. We set these in the Sources panel under the XHR/fetch Breakpoints section.
To add a breakpoint, we click the + button and enter a URL substring. DevTools will pause when a request URL contains that substring.
This allows us to inspect request and response data, modify headers, or step through the code that handles the response. It's particularly useful for debugging API interactions or investigating network-related issues.
Working with Event Listener Breakpoints
Event listener breakpoints pause code execution when specific events occur. We find these in the Event Listener Breakpoints section of the Sources panel.
To use them, we expand categories like Mouse, Keyboard, or Timer, and check the events we want to break on. For example, we might select the 'click' event to pause whenever a click occurs.
This helps us debug event-driven behaviors, inspect event objects, and understand how our code responds to user interactions. It's especially useful for tracking down issues in complex user interfaces or event-based systems.
Monitoring Network Activity
Chrome DevTools provides powerful tools for monitoring network activity during website loading. We can observe real-time requests, analyze performance metrics, and identify bottlenecks to optimize our web applications.
Understanding XHR and Fetch Calls
XHR (XMLHttpRequest) and Fetch are crucial for asynchronous data exchange in web applications. We can inspect these calls in the Network tab of Chrome DevTools.
To view XHR and Fetch requests:
- Open DevTools (Ctrl+Shift+J or Cmd+Option+J on Mac)
- Navigate to the Network tab
- Filter by XHR or Fetch
We can examine request headers, response data, and timing information for each call. This helps us debug API interactions and ensure efficient data loading.
XHR breakpoints allow us to pause script execution when specific XHR events occur. We can set these breakpoints to investigate request/response data at runtime.
Analyzing Load Performance and Bottlenecks
Chrome DevTools offers various metrics to evaluate load performance. We can identify bottlenecks by examining:
- Time to First Byte (TTFB)
- DOM Content Loaded
- Load time
The Network panel provides a waterfall view of resource loading. This visual representation helps pinpoint slow-loading resources.
To analyze performance:
- Enable the Network tab
- Reload the page
- Review the timeline and resource list
We can identify performance bottlenecks by looking for:
- Large file sizes
- Slow server response times
- Render-blocking resources
By optimizing these areas, we can significantly improve our website's load performance.